Mastering JUnit: A Comprehensive Training Guide
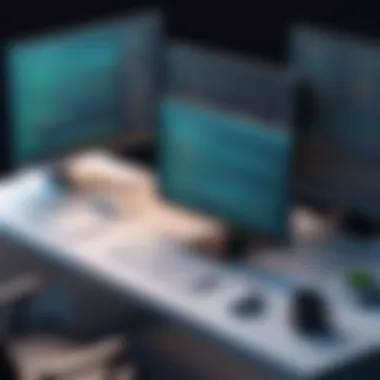
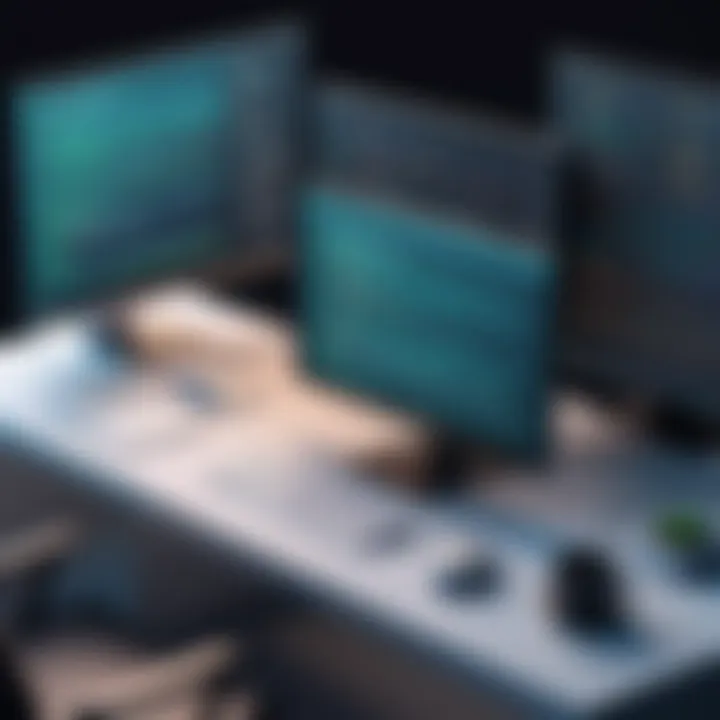
Intro
JUnit is an essential tool in the realm of Java programming. It facilitates the testing process of Java applications, which is crucial for ensuring software reliability and quality. In today’s fast-paced software development lifecycle, the effectiveness of JUnit cannot be overstated. Mastering JUnit can significantly streamline the testing workflow, making it a vital asset for both beginner developers and seasoned professionals alike.
Learning JUnit involves understanding its core functionalities, from installation to creating and executing tests. This guide aims to provide a structured approach, equipping readers with the necessary knowledge to optimize their JUnit experience.
Software Overview
JUnit is a framework designed specifically for unit testing in Java. It is part of the xUnit family of testing frameworks. JUnit offers a set of annotations and assertions that make it easier for developers to write and run tests.
Software Features
- Annotations: JUnit utilizes annotations like , , and to define test methods and manage test execution flow.
- Assertions: Assertions are used to verify that the outcomes of test cases are as expected. Common assertions include , , and .
- Test Suites: JUnit allows grouping of test cases into suites, facilitating the execution of multiple tests in one go.
- Integration with Build Tools: Works seamlessly with popular build tools like Maven and Gradle.
- IDE Support: Many Integrated Development Environments, such as IntelliJ IDEA and Eclipse, provide built-in support for JUnit, allowing direct execution of tests.
Technical Specifications
JUnit is primarily written in Java and requires a Java Development Kit (JDK) for functionality. The latest versions of JUnit are compatible with Java SE 8 and higher. JUnit 5 is the most current version, which introduces major enhancements and a more robust architecture compared to earlier versions.
JUnit is essential for maintaining high software quality by allowing developers to validate their code frequently and efficiently.
Peer Insights
User Experiences
Many developers have expressed that learning JUnit enhances their ability to write effective unit tests. Users often report that JUnit’s simplicity and extensive documentation make it easier to adopt. Developers find that integrating JUnit into their workflow results in fewer bugs and greater code reliability.
Pros and Cons
Pros:
- Improved code quality through rigorous testing.
- Extensive community support and documentation.
- Continuous updates and enhancements.
Cons:
- Might be overwhelming for complete beginners.
- Requires an understanding of testing concepts to use effectively.
As software development continues to evolve with agile methodologies, JUnit remains relevant, providing a robust solution for unit testing that aligns well with modern practices.
Prelims to JUnit
JUnit is an important framework in the Java ecosystem, primarily used for unit testing. This section will delve into the foundational elements that make JUnit a vital tool for software developers. It will cover an overview of JUnit, its significance in the software development process, and how it has evolved over time.
Overview of JUnit
JUnit is a testing framework that allows developers to write and run repeatable tests. Just like its name suggests, it is focused on unit testing, catering to the need for reliable, efficient, and prompt testing of individual parts of a software application. This framework is essential for ensuring that each component functions correctly in isolation. JUnit provides a simple and effective way to carry out tests and detect issues before they escalate into larger problems during the software development cycle.
Importance of Unit Testing
Unit testing is a critical aspect of software development. It provides a safety net for developers, ensuring that code changes do not introduce new defects. Testing individual units also promotes code quality and maintainability. When unit tests are run often, they can help identify problems early, thus saving time and resources. By adopting unit testing practices, teams can achieve higher productivity and confidence in their code.
"The cost of fixing a defect after development is significantly higher than identifying it during the unit testing phase."
Evolution of JUnit
JUnit has undergone significant changes since its inception. Initially released in 1997, it has evolved through multiple versions, each introducing enhancements that improve its functionality and general usability. Over the years, JUnit has adapted to modern development practices, integrating seamlessly with build tools and continuous integration frameworks. The evolution of JUnit mirrors the broader trends in software development, emphasizing the growing importance of automated testing and quality assurance. Developers today rely on JUnit as a robust tool that adapts to their changing needs and supports various methodologies in the agile landscape.
By understanding these initial aspects, learners can appreciate JUnit's role in facilitating high-quality software development. This sets the stage for deeper exploration into JUnit's functional capabilities, practical applications, and best practices for effective unit testing.
Setting Up JUnit
Setting up JUnit is a fundamental step in the journey towards effective unit testing in Java applications. This section aims to unravel the significance of configuring JUnit properly, ensuring a seamless experience throughout the testing phases. The necessity for precise setup cannot be overstated, as it lays the groundwork for future testing activities, impacting efficiency and reliability. Furthermore, understanding the system requirements, installation process, and integration with IDEs can greatly enhance productivity. By the end of this section, readers should feel equipped to successfully establish JUnit within their development environment.
System Requirements
Before the installation of JUnit, it is essential to assess the system requirements. First, ensure that Java Development Kit (JDK) is installed. JUnit operates on JDK version 8 or later. The Java Runtime Environment (JRE) may also be necessary, but typically, the JDK suffices for testing purposes.
Here are the key requirements:
- Operating System: Any OS that supports Java (Windows, macOS, Linux)
- IDE Compatibility: JUnit should work well with IDEs like IntelliJ IDEA, Eclipse, or NetBeans. Verify specific IDE requirements too.
- Memory: At least 1GB of RAM recommended for efficient running of build tools and IDEs.
- Disk Space: Sufficient space to accommodate installation packages and project files.
Being aware of these requirements can prevent headaches during installation and configuration phases.
Installation Process
Installing JUnit is typically straightforward. It can be integrated into your project as a dependency with build tools like Maven or Gradle, or it can be manually downloaded if desired.
Using Maven:
To use JUnit with Maven, add the following dependency in the :
This snippet specifies that JUnit is a testing dependency.
Using Gradle:
For Gradle, include this in the file:
Alternatively, if manual installation is preferred, navigate to the JUnit website, download the JAR files, and add them to your project library.
Configuring JUnit with IDEs
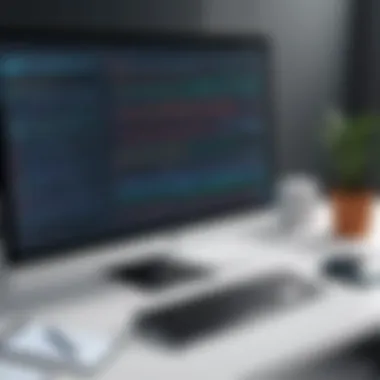
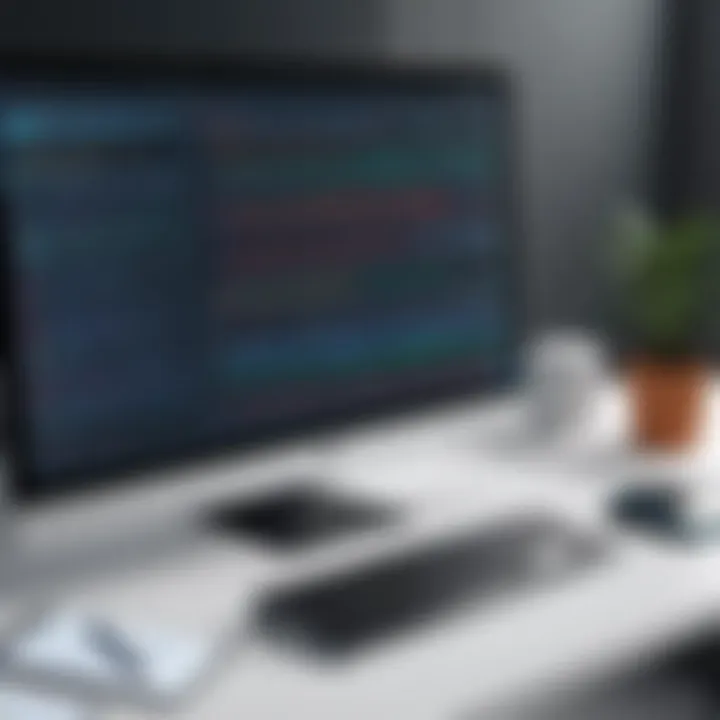
Once JUnit is installed, it's crucial to configure it with your Integrated Development Environment (IDE) for optimal use. Each IDE has a slightly different process for this setup, but the general principles remain the same.
- In Eclipse:
- In IntelliJ IDEA:
- Open Eclipse.
- Right-click on your project and select Build Path > Add Libraries.
- Choose JUnit and select the appropriate version.
- Open your project.
- Go to File > Project Structure.
- In the Libraries section, click on the + sign to add JUnit. Select the appropriate version and click OK.
Ensuring JUnit is properly configured in your IDE streamlines the process of writing and executing tests. This step enhances the development environment's readiness, contributing significantly to the robust testing framework that JUnit promotes.
Remember: Proper setup today lays the foundation for more insightful testing tomorrow.
By understanding these essential components, you can create a more effective and reliable testing framework that will benefit the entire development process.
Creating Your First JUnit Test
Creating your first JUnit test is more than just a technical task. It establishes a foundation for effective software testing and quality assurance. This section provides essential insights into the elements involved in writing JUnit tests, benefits derived from it, and various considerations to bear in mind.
Understanding Test Classes and Methods
In JUnit, a test class houses test methods that check the behavior of specific parts of your application. Understanding how to structure your test classes is critical for organized and maintainable code. A test class typically mirrors the structure of the class it tests. For example, if you have a class named , your test class could be named . This clear relationship makes it easier to locate tests associated with the application code.
A test method is usually annotated with , indicating that it is a unit test. Each method should focus on a single aspect of the behavior you want to test, enhancing clarity and precision in what the test covers. Naming conventions are also important; choose descriptive names that convey what is being tested. For example, a method named clearly indicates the intention of the test.
Using Annotations Effectively
Annotations are pivotal in JUnit as they provide metadata for test classes and methods. Several annotations can assist in managing the testing framework effectively:
- : This runs once before all tests in the class, suitable for costly setup tasks that are the same for all tests.
- : This is executed before each test method, ideal for creating a fresh state for each test.
- : This runs after each test, helpful for cleanup tasks.
- : This runs once after all tests, useful for shared resource deallocation.
Effectively utilizing these annotations results in cleaner tests and ensures that setup and teardown phases do not overlap or interfere with one another. This organization supports accurate tests and easier debugging.
Writing Your Initial Test
To write your initial JUnit test, start by creating a straightforward test case for a method in your functional class. Here's a concise example:
In this code, we define a test within the class. The test checks that the method in the class works correctly. The method verifies the expected outcome against the actual result. If the values don't match, JUnit will report the failure, indicating a problem that needs addressing.
Taking these steps will provide a practical start in writing your unit tests. The journey towards mastering JUnit begins with the precise and well-thought-out creation of your first tests.
Properly structured and clear tests lead to more dependable code and enhanced overall software quality.
As you grow in your ability to write tests, ensure that your tests remain focused, comprehensive, and maintainable. This solid foundation will serve you well as you delve deeper into the realm of JUnit testing.
Executing and Debugging Tests
Executing and debugging tests form a crucial part of the JUnit framework, serving as the bridge between writing tests and delivering high-quality software. These activities enhance the development process by ensuring that tests run efficiently and any defects are identified and resolved promptly. Understanding how to execute tests correctly impacts the pace of development and the reliability of results, while debugging acts as a necessary skill to uncover hidden issues within code.
Running Tests in IDE
Running tests within an Integrated Development Environment (IDE) simplifies the testing process, enabling developers to execute their JUnit tests seamlessly without command-line complications. Most popular IDEs like Eclipse, IntelliJ IDEA, and NetBeans provide built-in support for JUnit, offering features that streamline running and managing tests.
To run tests, developers typically look for the test class in the project pane and right-click to select the option to run. For example, in IntelliJ IDEA, right-clicking on the test file allows you to choose "Run 'TestName'". The IDE then compiles the code and executes the tests. This immediate feedback loop can be pivotal because it allows developers to assess whether their changes pass the tests before they commit their work.
Additionally, IDEs provide a visual representation of test results. Color-coded summaries indicate which tests have passed or failed, and stack traces help diagnose issues quickly.
Understanding Test Results
Analyzing test results is an essential skill for developers working with JUnit. After tests have executed, the results can reveal insights into the code's stability and expected functionality. Test results typically indicate whether a test has passed, failed, or encountered an error during execution.
When a test fails, JUnit outputs pertinent details, including:
- Failure message: It describes why the test did not succeed.
- Stack trace: This provides context on where the failure occurred, pointing developers to the exact line in the code that needs attention.
While reviewing results, developers should focus not only on passed tests but also on the failures. A consistent pattern of failing tests might signal underlying issues in the codebase that require corrective measures.
"Understanding test results is more than verifying functionality; it’s a pathway to improving code quality and confidence in the software."
Debugging Test Failures
Debugging is an integral part of the development process when working with JUnit. When a test fails, identifying the root cause is the next step in improving the code. Using built-in debugging tools, developers can step through the test's execution to inspect variable states, flow of control, and interaction among components.
In most IDEs, you can initiate debugging by setting breakpoints in the test or production code. Upon running the test in debug mode, the execution pauses at these breakpoints, allowing you to evaluate the state of the application down to the variable level. This step-by-step analysis helps to clarify why certain assertions may not hold true during run time.
Additionally, logging frameworks can assist in tracking the application behavior before tests fail. Logs provide a historical record of executions, offering context that IDE debugging alone might not reveal. Developers should adopt logging best practices, incorporating logs thoughtfully to avoid clutter and maintain clarity.
In summary, mastering test execution and debugging with JUnit not only enhances productivity but also supports ongoing software quality assurance. It enables developers to move confidently from coding to testing, ensuring that their applications meet user expectations.
Advanced JUnit Features
The domain of Continuous Integration and Test-Driven Development has evolved significantly. With this evolution, JUnit has introduced several advanced features that cater to more complex testing needs. Understanding these advanced features is crucial, as they provide developers with tools to enhance test efficiency and maintainability. This section discusses key elements such as parameterized tests, test suites, categories, and custom test runners. Each of these aspects presents unique benefits and considerations that can influence software quality management.
Parameterized Tests
Parameterized tests are an important feature in JUnit, allowing developers to run the same test multiple times with different inputs. This is highly beneficial for scenarios where functions accept various parameters to produce expected outcomes. Instead of writing multiple test methods, parameterized tests minimize redundancy and enhance code clarity.
By using this feature, you can define a test method once and provide it with different variable inputs. This makes it possible to easily see how the method behaves across various scenarios. For example, if testing a mathematical function, a developer could run the same test with different numbers to ensure broad coverage of possible cases.
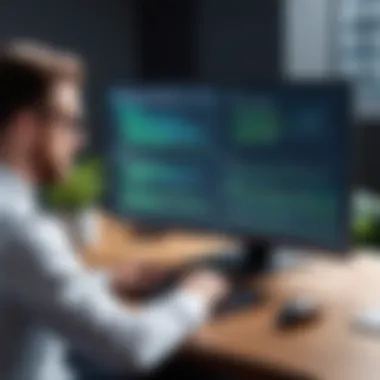
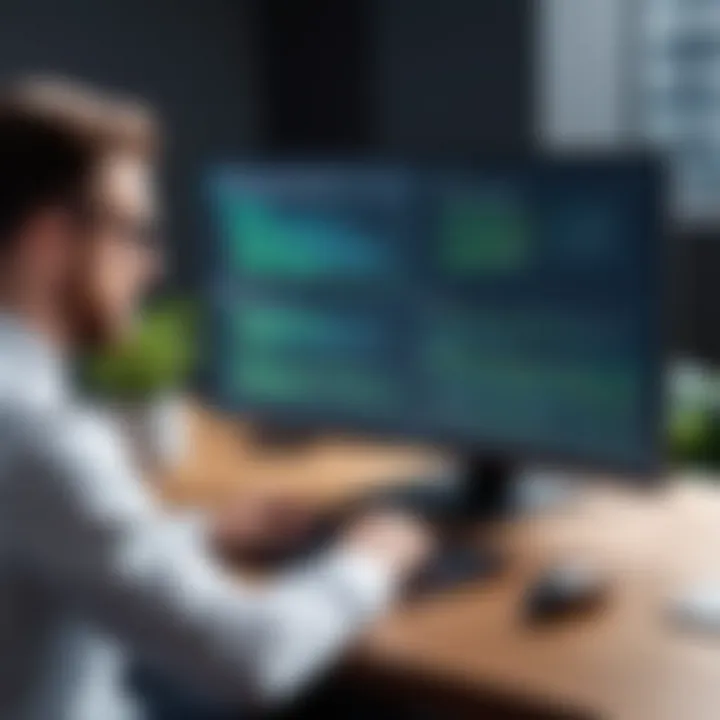
To implement a parameterized test, you can utilize the annotation in JUnit. This setup simplifies the testing process and allows for cleaner code. Here’s a simplified code example:
The above code provides a clear example of how parameterized tests can streamline testing processes, emphasizing both efficiency and clarity.
Test Suites and Categories
Test suites in JUnit allow users to group multiple test cases together for comprehensive execution. This organization improves test management and can significantly reduce time spent running tests, especially for large projects. By executing all relevant tests in a suite, developers can easily validate changes behind a feature or fix.
When you create a test suite, you can include specific test classes or individual tests. This granularity helps focus on certain aspects of the code without running the entire suite, therefore improving testing efficiency. Furthermore, categorizing tests into logical groupings aids in better management and execution. For instance, you could categorize tests for specific modules or features in your application.
A simple example of creating a test suite involves using the annotation as shown below:
This illustrates how to run multiple tests together, demonstrating JUnit's capability to streamline testing for larger projects.
Custom Test Runners
Custom test runners in JUnit empower developers to tailor the execution process according to specific requirements. A custom test runner can modify how tests are executed, providing more control over the testing lifecycle. This can include aspects such as handling test failures in unique ways or integrating with other tools.
Creating a custom test runner allows developers to encapsulate common behaviors for multiple tests. For example, if you want to log additional information before and after tests, a custom runner can handle this consistently across all relevant tests.
To implement a custom test runner, you need to extend the class and override the necessary methods. Here is a basic outline:
Such flexibility is vital for complex applications where standard testing frameworks might fall short.
In summary, these advanced JUnit features enhance the power of unit testing. By adopting them, developers can improve the quality of their tests while also increasing efficiency. As software continues to evolve, recognizing and utilizing these advanced tools will become increasingly important for maintaining robust application stability.
Integrating JUnit with Build Tools
Integrating JUnit with build tools is a critical aspect of enhancing testing efficiency in software development. Build tools such as Maven and Gradle automate the processes of compiling code, packaging binaries, and running tests. When JUnit is seamlessly integrated with these tools, it streamlines the testing phase within the development lifecycle. This integration not only reinforces coding standards but also allows for better management of dependencies and environments.
Using build tools ensures that tests are executed consistently and in the correct order after each build. This practice is essential for maintaining high code quality and facilitating continuous integration, which further allows teams to deliver reliable software quickly. Overall, integrating JUnit with build tools is a vital practice that benefits developers, testers, and the entire project workflow.
JUnit with Maven
Maven is a popular build automation tool used primarily in Java projects. Its declarative approach to project structure makes it easier to manage dependencies and build configurations. Integrating JUnit with Maven begins with ensuring JUnit is included as a dependency in the file.
Here is a basic example of how to add the JUnit dependency:
Placing this code in the allows Maven to download JUnit when building the project. Running tests is straightforward with Maven; simply executing the command triggers all tests defined in the project. This automation greatly reduces manual overhead and enhances collaboration within development teams.
JUnit with Gradle
Gradle is another widely adopted build automation tool that provides flexibility and performance benefits. Similar to Maven, JUnit can also easily integrate with Gradle. Developers add dependencies directly in the file.
A typical JUnit dependency in a Gradle build file might look like this:
With this setup, you can run your tests using the command. Gradle excels in performance due to its incremental build capabilities. This means that only changed files will be recompiled and retested, making it an efficient solution for large codebases.
JUnit with Continuous Integration Tools
Continuous Integration (CI) tools like Jenkins, CircleCI, and Travis CI play a vital role in modern software development practices. Integrating JUnit with these CI tools allows automated execution of tests every time code is pushed to a repository. This ensures that any issues are promptly identified, facilitating a rapid feedback loop.
When setting up CI pipelines, it is essential to configure the build tool (Maven or Gradle) to run JUnit tests as part of the build process. A typical CI configuration includes steps where the code is checked out, dependencies are resolved, and tests are executed.
For example, a simple Jenkins pipeline configuration for running JUnit tests might include:
This integration guarantees that the software’s reliability is tested continuously throughout the development process. By adopting automated testing with CI tools and JUnit, teams can substantially decrease the time it takes to determine the quality of their code.
Best Practices for JUnit Testing
Best practices in JUnit testing are essential for developing high-quality software. They help developers create effective unit tests that are easy to read, maintain, and execute. Following these practices can lead to a more efficient development process and improved software reliability. Here, we focus on key aspects like readability, test coverage, and handling exceptions within tests.
Maintaining Readability and Simplicity
Readability in test cases is crucial. Well-written tests should be understandable at a glance. This encourages collaboration among team members and simplifies debugging. To ensure simplicity, consider the following:
- Descriptive Names: Use specific names for test methods. For example, instead of , use . This immediately informs the reader of the test's purpose.
- Single Responsibility Principle: Each test should focus on a single behavior. This way, if a test fails, it’s clear what went wrong.
- Avoid Complexity: Keep test logic straightforward. If a test involves multiple conditions, break it into several simpler tests. This approach gives clarity and prevents confusion.
These practices enhance understanding and allow quick identification of issues.
Test Coverage and Its Importance
Test coverage measures how much of your code is tested by unit tests. High coverage indicates a greater likelihood of catching defects before deployment. However, achieving high coverage is only part of the equation. Quality matters more than quantity. Here are some points to consider:
- Focus on Critical Paths: Prioritize testing the key functionalities of your application. Critical paths are where most defects occur.
- Identify Gaps in Coverage: Use tools like JaCoCo to identify untested parts of your code. Regular tracking of coverage prevents neglecting vital areas.
- Balance Between Unit and Integration Tests: While unit tests should cover individual components, integration tests ensure that those components work together. A blended approach provides a robust testing strategy.
Maintaining an appropriate level of test coverage enhances confidence in the software's reliability.
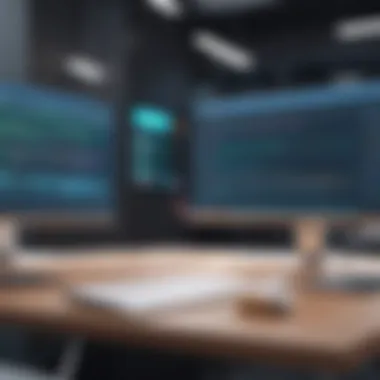
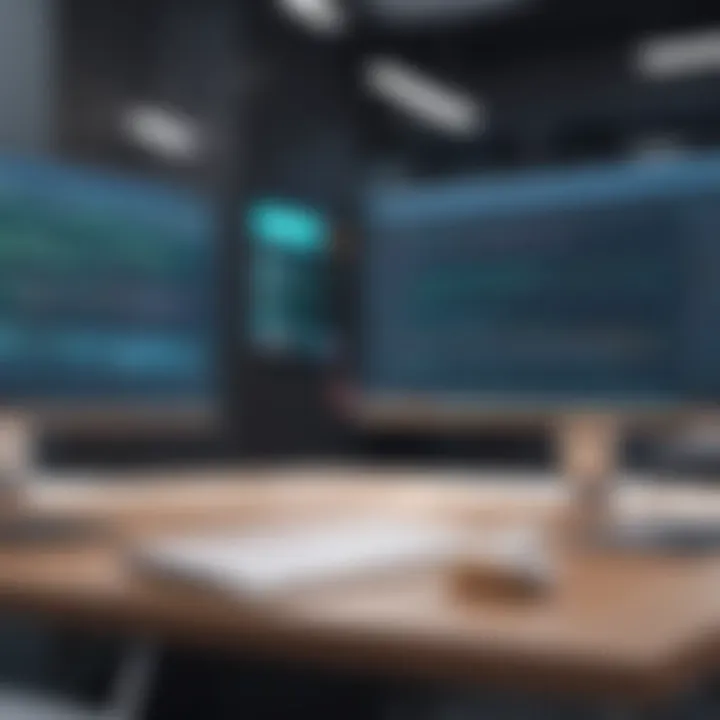
Testing Exceptions and Edge Cases
Testing exceptions and edge cases is vital for building resilient applications. Many programs work correctly under normal conditions but fail when faced with unexpected inputs or situations. To manage this, developers should:
- Anticipate Edge Cases: Think of unusual input that might cause failure. For instance, what happens if a function receives a null value?
- Use appropriate Assertions: Utilize JUnit assertions like to verify that exceptions are thrown in the expected scenarios. Example:
- Test Resilience: Consider not just the happy path but also failure scenarios. Applications should respond gracefully to various failure conditions.
By incorporating these practices, developers create tests that protect against unexpected behaviors and improve the overall software quality.
"Well-structured tests not only catch bugs but also communicate the intended behavior of the code."
Employing best practices in JUnit testing leads to an organized and effective testing suite that enhances software maintainability and reliability in the long run.
JUnit in Agile and DevOps
In the dynamic landscape of software development, the role of JUnit in Agile and DevOps practices becomes particularly significant. Agile methodologies emphasize iterative development, collaboration, and adaptive planning. Consequently, the integration of unit testing using JUnit stands as a critical element to ensure consistent quality in this fast-paced environment. As teams adopt Agile and DevOps practices, the need for reliable testing frameworks like JUnit arises to promote a seamless flow from development to deployment.
Role of JUnit in Agile Development
JUnit plays a pivotal role in Agile development by facilitating continuous integration and delivery. It allows developers to write tests concurrently with the production code, ensuring that every feature is validated early in the development cycle. This not only enhances test coverage but also limits the accumulation of technical debt, which can arise from rushed or incomplete testing.
Moreover, by enabling immediate feedback, JUnit promotes a culture of accountability among team members. When a test fails, developers have clear visibility into the malfunctioning component of their code, leading to prompt resolutions. Engaging with JUnit in an Agile context enhances the overall reliability of the software and aligns well with Agile principles such as Responding to Change and Customer Collaboration.
Continuous Testing Practices
In Agile and DevOps environments, continuous testing is essential. This practice integrates JUnit as a vital tool for ensuring software quality at every stage of the development cycle. Continuous testing with JUnit means that tests are executed frequently, often with each code commit.
Using JUnit in this manner allows for rapid validation of new features, ensuring they behave as expected before further integrations. Moreover, with Continuous Integration tools, these tests are automated. This automation means that teams can run hundreds or thousands of tests with minimal human intervention, saving time and reducing errors.
The main benefits of continuous testing with JUnit include:
- Immediate Feedback: Detect issues as they arise, rather than later in the cycle.
- Increased Test Coverage: Encourages thorough testing of new and existing features.
- Cost Efficiency: Reduces costs associated with late-stage defect detection.
Feedback Loops and Iteration
Feedback loops are crucial in Agile and DevOps, serving as mechanisms to ensure that teams can quickly implement improvements based on testing outcomes. JUnit fosters these feedback loops by providing detailed reports on test results.
When developers run tests, they receive immediate feedback about their code’s performance. This helps in identifying patterns, such as frequently failing tests or problematic areas in the codebase. Iterating based on this feedback leads to more refined code and allows teams to ensure that user expectations are met.
Iterative cycles support enhancements in product quality over time. By analyzing test failures, teams can determine whether the failure is due to a bug, a misunderstanding of requirements, or an issue with the test itself. Ultimately, the integration of JUnit leads to better iterations and enhancements in both product reliability and functionality.
"Integrating JUnit into your Agile or DevOps processes not only streamlines testing but fundamentally enhances your collaborative development efforts."
Adopting JUnit within Agile and DevOps practices creates a more resilient development process that prioritizes quality assurance and rapid iteration, aligning perfectly with the ethos of modern software development.
Future Trends in Testing
In today's rapidly evolving software landscape, understanding the future trends in testing is crucial for professionals who aim to enhance their software quality assurance processes. Testing is not a standalone activity; it is deeply integrated into the development lifecycle. As technology advances, so do the methodologies used in testing. Being aware of emerging trends can lead to more efficient processes and higher-quality software.
Emergence of Automated Testing
Automated testing is becoming the standard in software development. The main advantage of automated testing is speed. Tests that run manually can delay development significantly. Automated testing reduces this time, allowing teams to respond to changes more rapidly. Tasks that were once repetitive and tedious are now handled by scripts or programs.
- Benefits of Automated Testing:
- Consistency: Automated tests are run the same way every time.
- Efficiency: They save time, especially in regression testing.
- Scalability: Tests can be executed on multiple configurations simultaneously.
Automated testing frameworks, such as JUnit, provide developers with tools to create comprehensive test suites. As automated testing becomes more common, integrating it into existing workflows is essential. Fabricating robust automated tests not only decreases human error, but also helps in maintaining high code quality during continuous integration.
Impact of AI on Testing Methodologies
Artificial intelligence (AI) is reshaping many sectors, and software testing is no exception. AI has the potential to enhance testing methodologies significantly. From test case generation to predicting areas of the application that may cause failures, AI is becoming a pivotal part of the testing landscape.
"AI technologies help in predictive analysis, optimizing testing efforts by identifying weaknesses in code."
- Key impacts of AI include:
- Intelligent test case management based on past failures and successes.
- Automated anomaly detection in application behavior.
- Continuous learning from test outcomes to optimize future testing cycles.
Utilizing AI in testing allows teams to bridge the gaps in current methodologies, especially concerning the efficiency and accuracy of test executions. This change not only accelerates the testing process but also leads to better resource allocation.
JUnit's Adaptation to New Technologies
As technologies evolve, JUnit has also adapted to stay relevant. New features and integrations ensure that JUnit remains a favorite among developers. The library continues to improve by incorporating support for emerging trends, such as automated tests and integration with AI-driven testing tools.
- Recent adaptations of JUnit include:
- Support for parameterized tests, allowing a single test method to run with multiple inputs.
- Enhanced integration with modern build tools like Maven and Gradle to streamline handling of dependencies.
- Improved annotations that allow for more readable and maintainable test code.
End
The conclusion serves as a critical element in this article, encapsulating the significance of mastering JUnit for effective software testing. As we have explored various facets of JUnit, from its setup to advanced features, this section will highlight the essence of what has been discussed. Informed understanding of JUnit enables developers and testers to enhance code quality and maintainability. It is not just a tool but a framework that integrates seamlessly into the development process, ensuring that software meets its functional requirements.
Summary of Key Points
Reflecting on the prior sections, here are the key takeaways:
- JUnit Overview: Understanding what JUnit is and its historical significance in software testing.
- Setting Up JUnit: Proper installation and configuration within different IDEs are mandatory for efficient operation.
- Test Creation: Annotations and structured test classes are crucial for writing effective test cases.
- Execution and Debugging: Running tests effectively and troubleshooting failures form the backbone of a strong testing approach.
- Advanced Features: Utilizing parameterized tests and custom test runners can significantly improve testing capabilities.
- Integration: Understanding how JUnit works with build tools like Maven and Gradle is essential for streamlined development.
- Best Practices: Upholding readability, ensuring test coverage, and focusing on edge cases leads to robust testing routines.
- Agile and DevOps: JUnit's role in modern methodologies enhances collaborative development and continuous integration practices.
- Future Trends: Awareness of emerging trends in automated and AI-driven testing results in preparedness for the evolving landscape.
Next Steps in Learning JUnit
To further deepen your understanding of JUnit, consider the following steps:
- Practice: Consistent hands-on experience is invaluable. Create various types of tests with different configurations.
- Explore Documentation: Leverage resources such as the official JUnit documentation and community forums on Reddit to stay updated.
- Join Community Discussions: Engaging in conversations with peers or forums like Stack Overflow can provide insights and solutions to common issues.
- Advanced Courses: Look for online courses that delve into advanced topics in JUnit, often available on platforms like Udemy or Coursera.
By pursuing these steps, not only will you solidify your understanding of JUnit, but you will also position yourself for success in developing high-quality software solutions.